All Virtual Methods Are Abstract in CPP
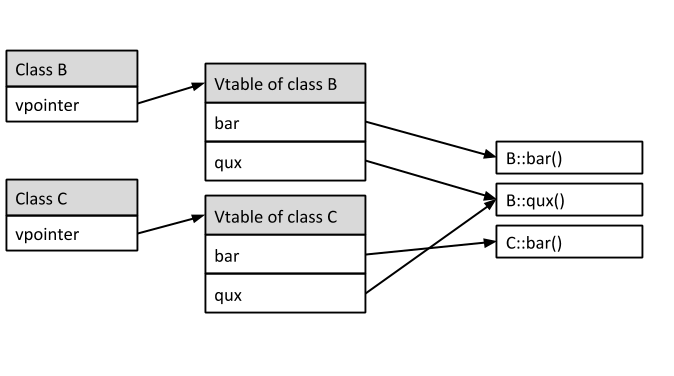
Virtual is a keyword to declare that the method must be override
If all method is virtual and equals 0, the class runs as an interface.
Override keywords shows that the virtual method has been overridden.
The shared pointer is the smart pointer way to declare a variable as a pointer. You also use a unique pointer too.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> #include <memory> class IParent{ public: // all fuctions are virtual // as a result // this class is abstract. virtual void vmethod() = 0; }; class MyChild : public IParent{ public: // all virtual method must be overreded // with override keyword void vmethod() override{ std::cout << "vmethod()" << std::endl; }; }; int main() { // only shared pointers may use on abstract std::unique_ptr<IParent> p { new MyChild{}}; p->vmethod(); return EXIT_SUCCESS; } |
The base classes deconstruction method should be virtual every time. Otherwise, its deconstruction doesn’t work in polimofic cases.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
#include <iostream> #include <memory> class MyParent{ public: MyParent(){ std::cout << "MyParent()" << std::endl; } virtual ~MyParent(){ std::cout << "~MyParent()" << std::endl; } }; class MyChild : public MyParent{ public: MyChild(){ std::cout << "MyChild()" << std::endl; } ~MyChild(){ std::cout << "~MyChild()" << std::endl; } }; int main() { // only shared pointers may use on abstract std::unique_ptr<MyParent> p { new MyChild{}}; return EXIT_SUCCESS; } |
You can not override the inherited method if it is not mentioned by virtual keyword. If a class not suitable for inheriting we should mark it with final keyword.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
class MyClass final{ public: MyClass(){ std::cout << "MyClass()" << std::endl; } //it cant be inherited because it is not //an virtual method void method(); //deconstruction is not virtual causeses memory leak ~MyClass(){ std::cout << "~MyClass()" << std::endl; } }; |