Template
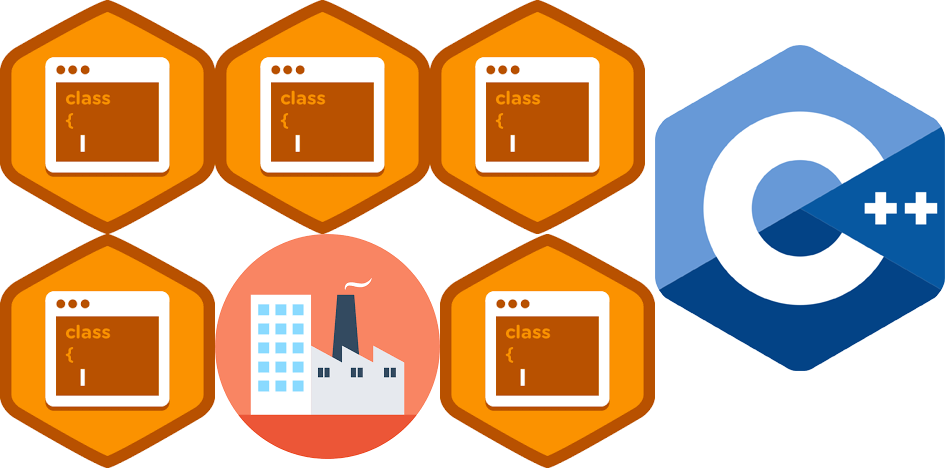
Here you are a short review code for templates
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
#include <iostream> template<typename T> T maxFunc (T x, T y){ std::cout << typeid (T).name() << " maxFunc (" << typeid (T).name() << " x, " << typeid (T).name() << " y)" << "("<< x << "," << y << ")" << std::endl; return x > y ? x : y; } // Explicit Initialization template char maxFunc(char x, char y); // Explicit Specialization template<> int * maxFunc (int * x, int * y ){ std::cout << "template<> int * maxFunc (int * x, int * y )" << "(" << *x << "," << *y << ")" << std::endl; int * ret; ret = *x > *y ? x : y; return ret; } //template argument template <int i> void doprint(){ std::cout << "Doprint" << i << std::endl;s } int main() { std::cout << maxFunc(3,2) << std::endl; std::cout << maxFunc<double>(3.2,2) << std::endl; int * a {new int}; int * b {new int}; *a = 2; *b = 3; std::cout << *maxFunc(a,b) << std::endl; doprint<2>(); return EXIT_SUCCESS; } |
Explicit Initialization forces compiler to create a version of code.
Explicit Specialization is the way for creating an alternative implementation of a template method.