To be Modern or Not
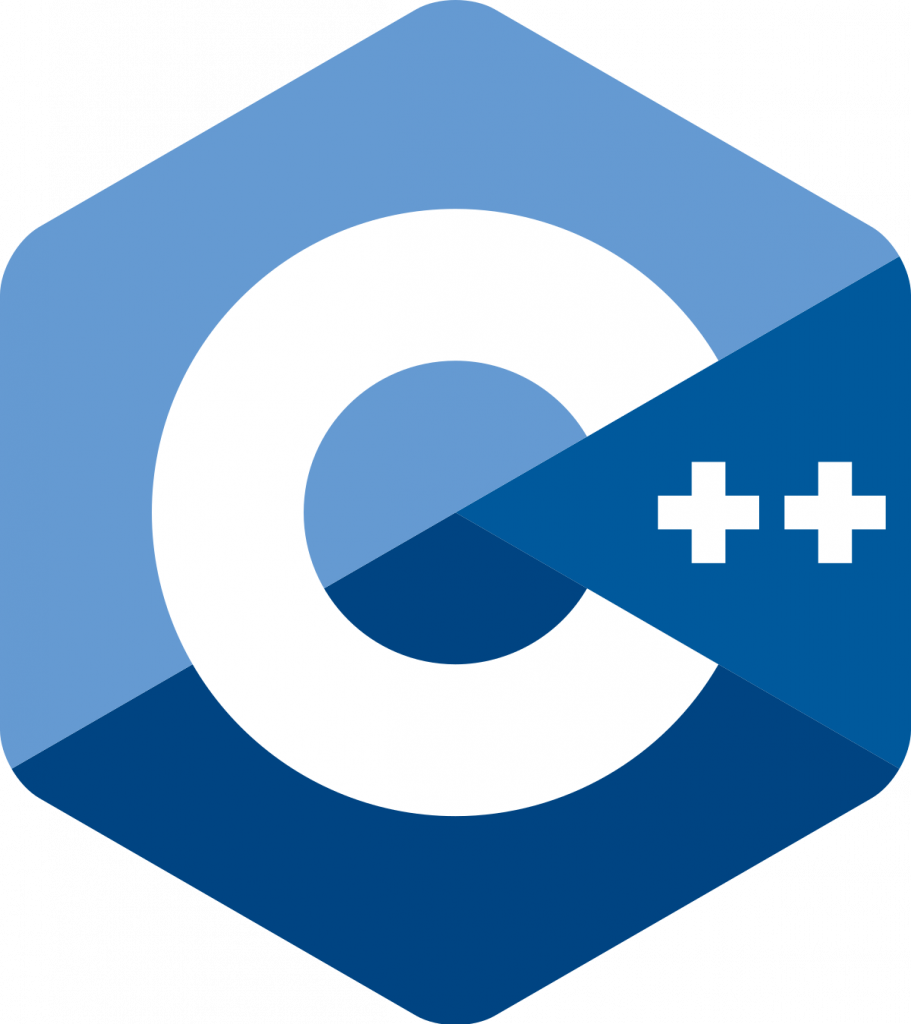
C++ has great change after C++11 and it still continues. I shared some of the basic important topics before. But I wish to add a summary here ;
- Use nonstatic data initialization instead of the old way. (Why nonstatic data initialization is important)
- Delete unused constructors with delete keyword.
- If you wish your compiler overcome a constructor method, Default keyword helps you
- Delegating parameters to parent method or a term which is a member of the class so easy in modern.
- Every time try to provide copy constructors.
- Copy constructor not enough! add a move constructor.
- std::move is your new helper.
- Never use c-type casketing.
- Always use smart pointers.
- Prefer unique_ptr if your pointer won’t be to pass an argument
- Shared_ptr is the best way if you pass a pointer.
- Use weak_ptr to reach counter pointer of shared pointers.
- Use enum class instade of the old enum type.
- To create a function which runs on compile-time learn constexpr
- Initializer_List basic list type which is generated by the compiler.
- using keyword is the way to implement constructors of inheritance.
- Default virtual methods are 0.
- Write all method in virtual if you wish to overload on the child.
- Final is the way to stop this override process.
- Never forget the overload keyword.
- Virtual classes important on Dimond inheritance
- You can’t throw an exception from deconstruction method.
- Template is important
- Non-type templates include the size of arrays
- Learn how to forward a parameter
- The variadic template uses stf:forward
- Lambda is everyting
- Lamdas are function objects
- Learn concurrency
- Mutex is the way to protect variable
- Atom is the new memory model
I hope it helps