Perfect Forwarding with Template
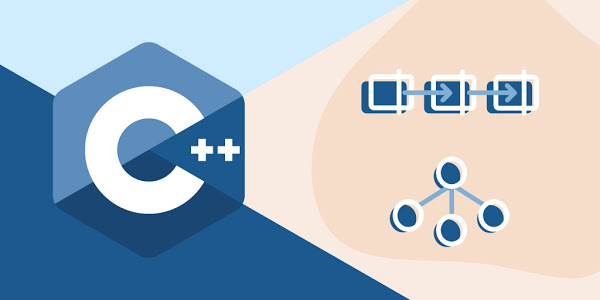
By writing a constructor template you can cover several alternatives of the constructors.
But there is also a way to call copy constructor or move constructor when you forwarding an object.
In flowing code, we provided a Has-A type class to understand what type of constructor called.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
#include <iostream> #include <string> class HasAClass{ std::string m_name; public: HasAClass() : m_name({}){ std::cout << "HasAClass()" << std::endl; } HasAClass(const std::string &name) : m_name(name) { std::cout << "HasAClass(const std::string &name)" << std::endl; } //a move based constructor HasAClass(std::string &&name) : m_name(std::move(name)) { std::cout << "HasAClass(std::string &&name)" << std::endl; } HasAClass(const HasAClass &p ) : m_name(p.m_name) { std::cout << "HasAClass(const HasAClass &p )" << std::endl; } // a basic move forwarding HasAClass(HasAClass &&p) : m_name(std::move(p.m_name)) { std::cout << "HasAClass(const HasAClass &&p )" << std::endl; } ~HasAClass(){ std::cout << "~HasAClass()" << std::endl; } }; class MyClass{ HasAClass h_c; public: //creates all alternatives template<typename T> MyClass(T &&s) : h_c(std::forward<T>(s)){ std::cout << "T is " << typeid (T).name() << std::endl; } }; int main() { // for move construct MyClass m_c1{"Move"}; // for copy constructor std::string s{"Copy"}; MyClass m_c2{s}; //has a class move constructor MyClass m_c3{HasAClass{"has-a-class"}}; //has a class copy constructor HasAClass ha_c{"has-a-class-copy"}; MyClass m_c4{ha_c}; } |
In the Has-A class, we implemented 4 types of initialization 2 for copy and 2 for the move. You may mention that std::mode has been used to forward parameter without creating a copy of it.
Template method have been used on the MyClass Class and it uses std:: forward method.
std::forward is a template method which looks the type of typetypes. If the type of the template is a reference type, it creates a copy of the object, otherwise moves the object.
1 Response
[…] option is reading. Yes, it is a very new blog I know but if you want you can read my post such as Perfect Forwarding with Template to dive in C++11/14-17 or […]