Lambda
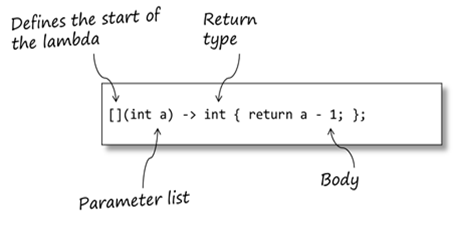
Lambda is a method to create a function object in C++. The basic structure is:
1 |
[<symbol>](<args>)<mutable><exeption_spec> -> <return_type> {//codes} |
To get the type of the lambda;
1 2 3 4 5 6 7 |
#include <iostream> int main() { auto fn = [](){return 0;}; std::cout << typeid (fn).name() << std::endl; } |
You may mention that lambda is a function object.
If you using C++14 or letter. you can use auto keyword to create template lambda. To change your Cmake setting please check the flowing line.
1 |
set(CMAKE_CXX_STANDARD 14) |
and the using auto on C++
1 2 |
auto fn = [](auto x){return x * x;}; std::cout << fn(2) << std::endl; |
importing a variable as copy
1 2 3 4 5 6 7 8 |
#include <iostream> int main() { int veriable = 3; auto fn = [veriable](auto x){return veriable * x;}; std::cout << fn(2) << std::endl; } |
the way to mutate a capture item
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <iostream> int main() { int veriable = 3; // mutable mutate the data but not original capture item auto fn = [veriable](auto x) mutable{ veriable++; return veriable * x; }; std::cout << fn(2) << std::endl; std::cout << "veriable" << veriable << std::endl; } |
the best way to mutate variable out of the scope is using referance capture item.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <iostream> int main() { int veriable = 3; // mutable mutate the data but not original capture item auto fn = [&veriable](auto x){ veriable++; return veriable * x; }; std::cout << fn(2) << std::endl; std::cout << "veriable " << veriable << std::endl; } |
To use all variables in the scope and also global just use & sign in capture list f lambda
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <iostream> int main() { int veriable = 3; // mutable mutate the data but not original capture item auto fn = [&](auto x){ veriable++; return veriable * x; }; std::cout << fn(2) << std::endl; std::cout << "veriable " << veriable << std::endl; } |
And = for using copies.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <iostream> int main() { int veriable = 3; // mutable mutate the data but not original capture item auto fn = [=](auto x) mutable{ veriable++; return veriable * x; }; std::cout << fn(2) << std::endl; std::cout << "veriable " << veriable << std::endl; } |